Mastering Kotlin Coroutines: Choosing Between Default and IO Dispatchers for Optimal Performance
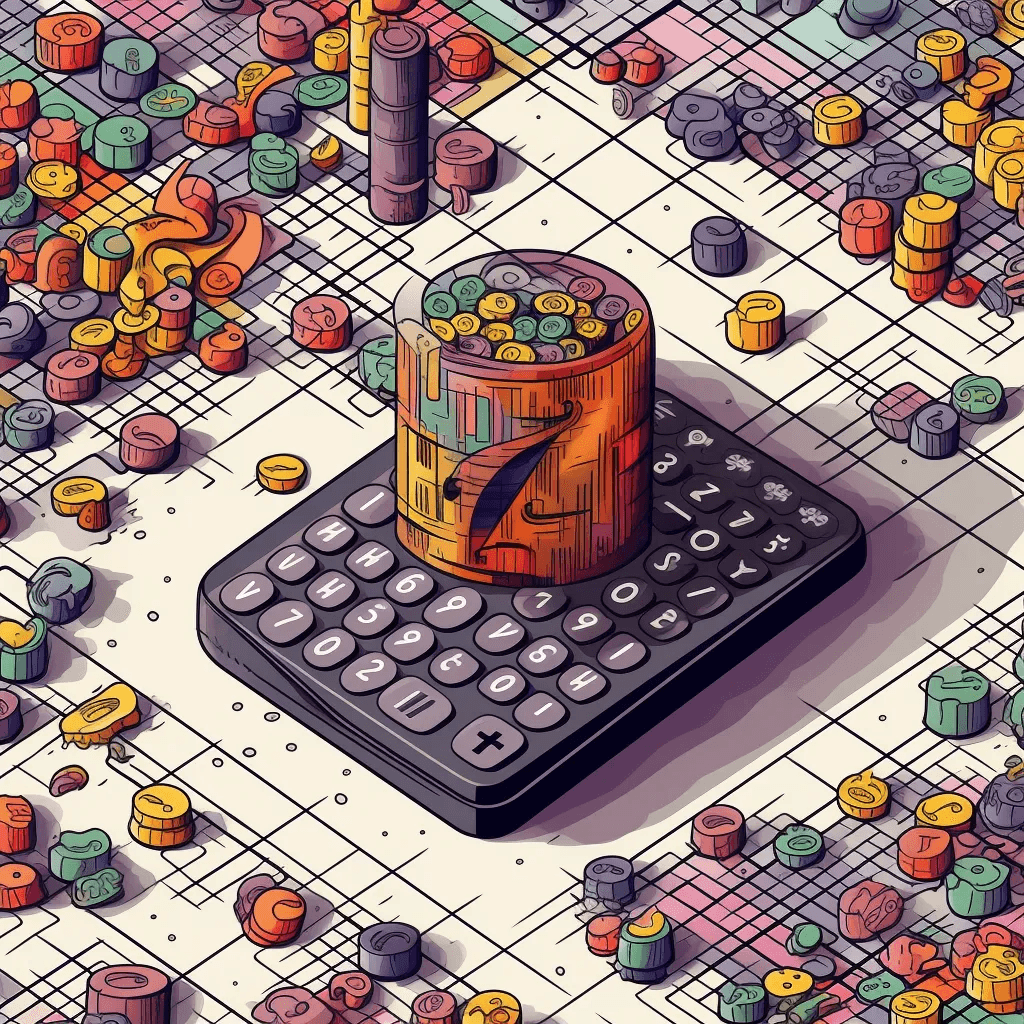
Kotlin Coroutines have become a powerful tool for writing asynchronous and concurrent code. Choosing the correct coroutine dispatcher is essential for achieving optimal performance and responsiveness when working with coroutines. In this article, we will explore the differences between two commonly used coroutine dispatchers: Default
and IO
. We'll discuss their characteristics and suitable use cases and provide real-life examples to help you make informed decisions when selecting a dispatcher for your coroutines.
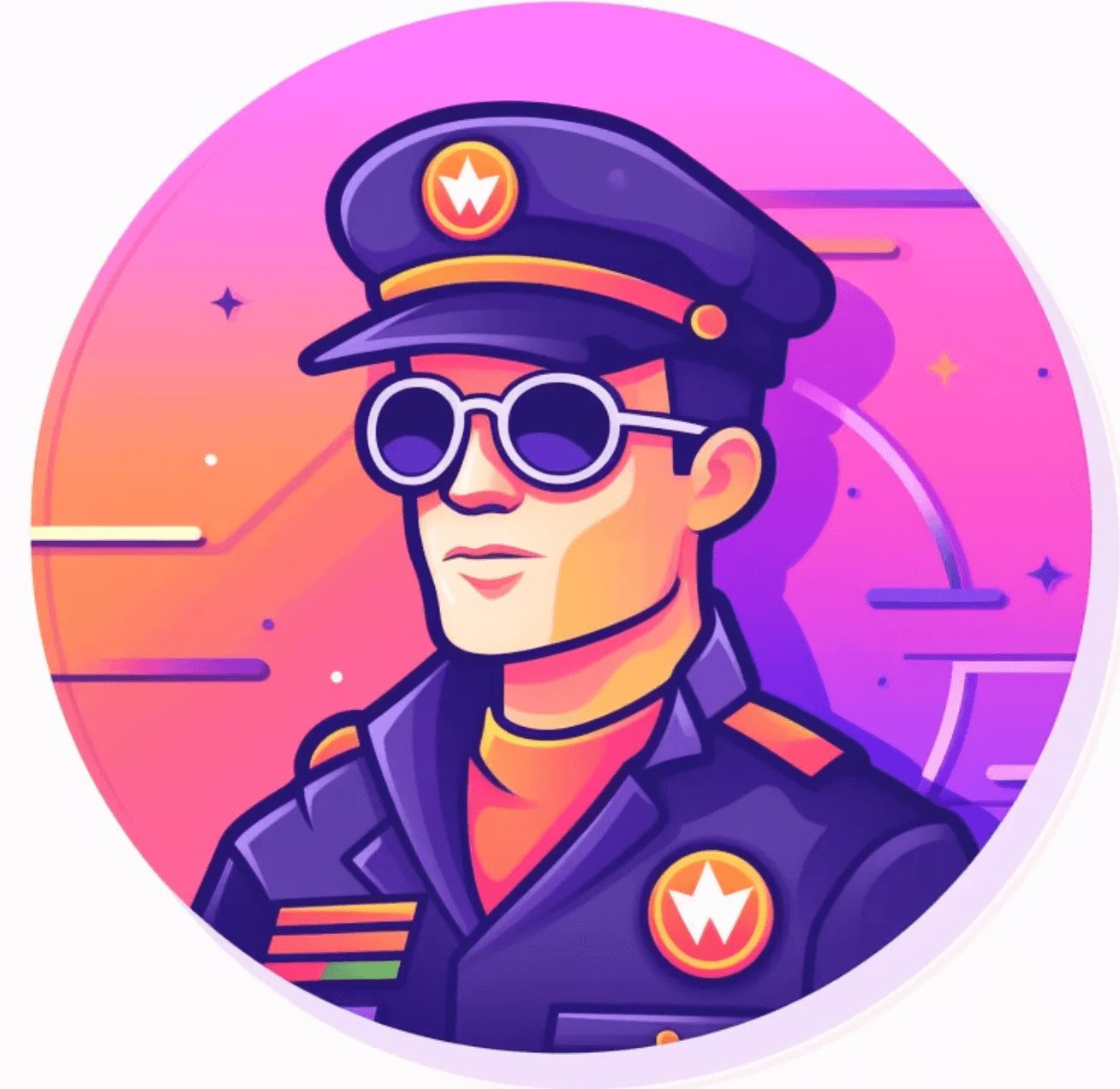
Default Dispatcher
The Default
dispatcher is designed for CPU-bound tasks that do not involve blocking I/O operations. It provides a limited number of threads equal to the number of CPU cores, enabling parallel execution of computationally intensive tasks. Here are some key points about the Default
dispatcher:
Usecases
- Computation-intensive tasks: Complex mathematical calculations, image processing, or heavy data transformations benefit from parallel execution using the
Default
dispatcher. - CPU-bound algorithms: Sorting, searching, graph traversal, or other algorithms that heavily rely on CPU resources can leverage the
Default
dispatcher for improved performance. - Encryption/Decryption: Cryptographic operations involving large files or multiple tasks can take advantage of the parallel processing capabilities of the
Default
dispatcher.
Real-Life Examples
- Image/Video Processing: Resizing, filtering, or transcoding images or videos can be done efficiently using the
Default
dispatcher. - Data Transformations: Complex data aggregations, statistical analysis, or machine learning algorithms can benefit from parallel execution provided by the
Default
dispatcher. - Computational Modeling: Physics simulations, weather forecasting, or financial modeling tasks involving heavy computations can be distributed across multiple threads with the
Default
dispatcher.
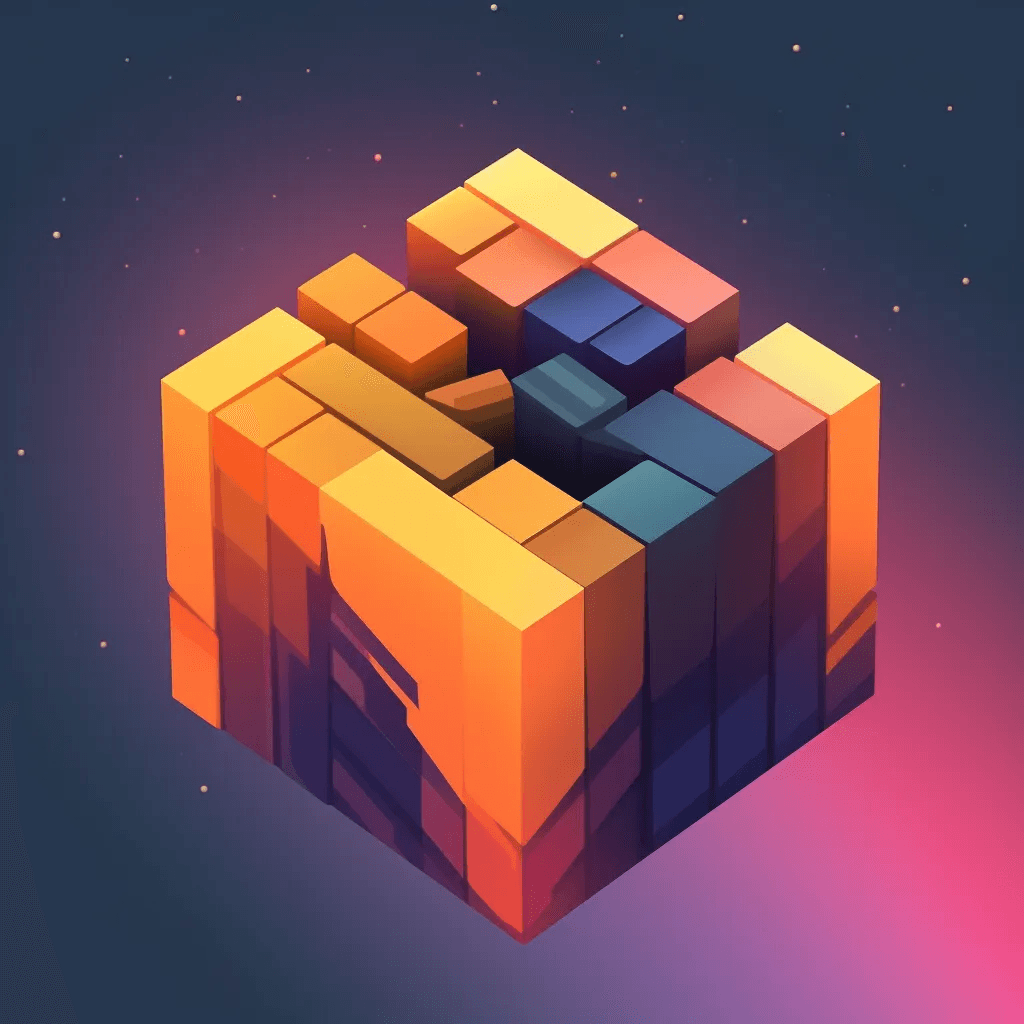
IO Dispatcher
The IO dispatcher
is optimized for tasks involving I/O operations, such as network requests, file operations, or database interactions. It is designed to handle blocking I/O operations efficiently by utilizing a larger thread pool. Here are some key points about the IO dispatcher:
Use Cases
- Network Operations: Making network requests to fetch data from APIs, downloading files or *uploading data are suitable use cases for the
IO dispatcher
. - File Operations: Reading from or writing to files, including file system operations or processing large datasets stored in files, benefit from the
IO dispatcher
. - Database Operations: Performing database queries, inserts, updates, or deletes should be done using the
IO dispatcher
for efficient I/O-bound execution.
Real-Life Examples:
- REST API Calls: Making HTTP requests to external APIs, such as fetching data or sending data to a remote server, should use the
IO dispatcher
to avoid blocking the main thread. - Database Queries: Performing database operations like queries, updates, or inserts should utilize the
IO dispatcher
for efficient I/O execution. - File Upload/Download: Uploading or downloading files from remote servers or cloud storage services should use the
IO dispatcher
to handle the I/O operations concurrently.
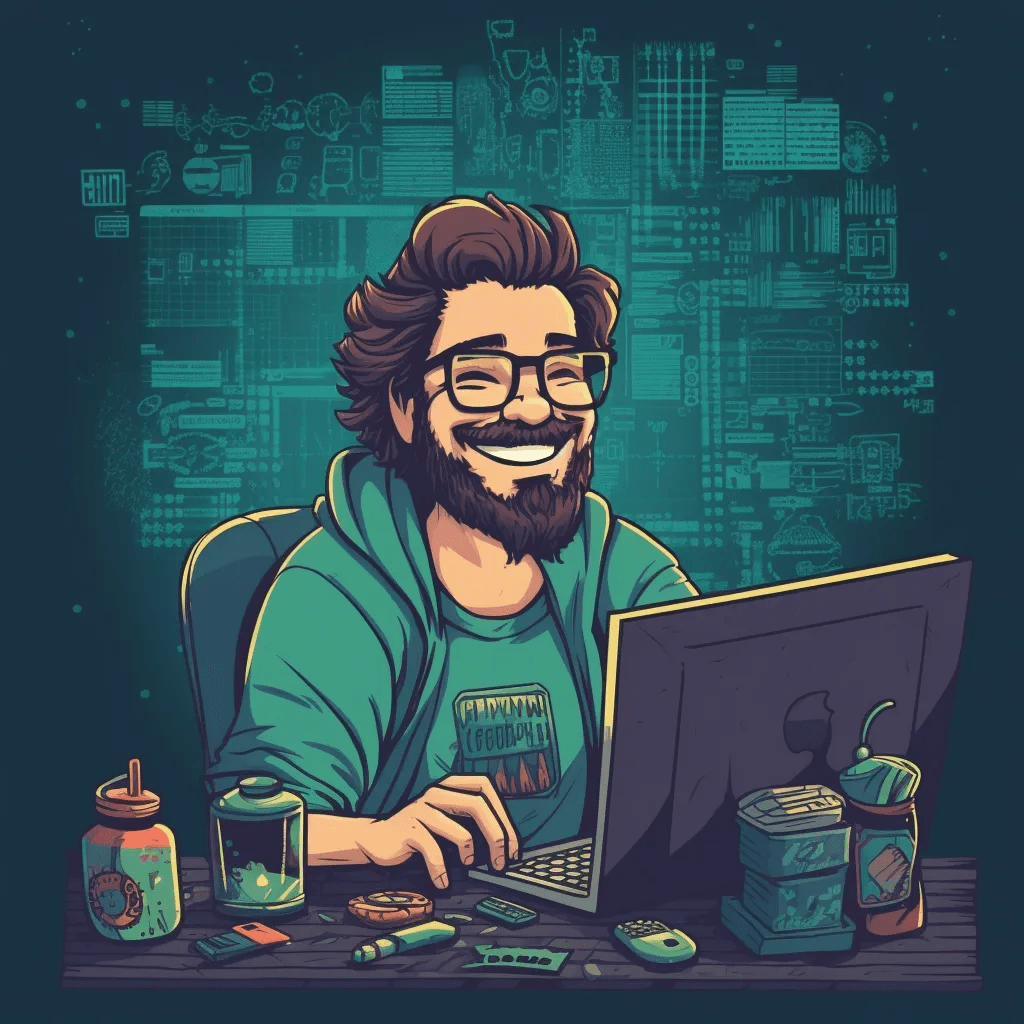
Conclusion
Choosing the appropriate coroutine dispatcher is crucial for ensuring the performance and responsiveness of your Kotlin Coroutines. The Default
dispatcher is ideal for CPU-bound tasks and computationally intensive operations, while the IO dispatcher
is optimized for I/O-bound operations such as network requests, file operations, and database interactions. By understanding these dispatchers' characteristics and suitable use cases, you can make informed decisions and leverage the full potential of Kotlin Coroutines.
Remember, these guidelines are not strict, and you may encounter scenarios requiring a different approach. Always measure and evaluate the performance of your coroutines to make informed decisions.
Thank you for reading! I hope you found this post helpful. Please consider sharing it with your friends and colleagues if you enjoyed it. You can also follow me on LinkedIn or Twitter to stay up-to-date on my latest posts. As always, I welcome your feedback and comments. Thank you again for your support!